|
|
WPF - Creazione dei controlli immagini |
 |
|
Creare controlli immagini scalabili per WPF |
|
Creare controlli immagini scalabili in WPF è una procedura che si suddivide in 4 passaggi fondamentali:
- Trasformare un'immagine vettoriale (svg o altro formato) in codice XAML
- Creare il controllo che gestisca lo XAML
- Allegare lo XAML al progetto
- Includere il controllo nelle nostre pagine XAML
|
Procediamo quindi con ordine e vediamo come trasformare un'immagine da svg a XAML.
Esistono numerosi strumenti anche sul web che permettono di creare XAML da una immagine SVG, ma io preferisco utilizzare Inkskape che รจ gratuito, opensource e reperibile qui: inkscape.org
- Una volta installato Inkscape (non è qui che andremo ad imparare ad utilizzare Inkscape), selezionare il comando File > Apri e scegliere l'immagine che si vuole aprire.
- Se si vogliono apportare delle modifiche al disgno
- Selezionare il comando File > Salva come
- Dalla finestra che si apre, selezionare il formato "Microsoft XAML (.xaml)"
- Spuntare la voce "compatibile Silverlight" se compare.
A questo punto abbiamo un file .xaml che contiene tutto il disegno che useremo successivamente.
|
Ora lavoriamo in Visual Studio. Nella nostra soluzione, se non già, creiamo una nuova cartella "Controls" e dentro aggiungiamo una nuova class.
[ContentProperty(ImageContentPropertyName)]
public class BasketCourtCtrl : Control
{
static BasketCourtCtrl()
{
DefaultStyleKeyProperty.OverrideMetadata(typeof(BasketCourtCtrl), new FrameworkPropertyMetadata(typeof(BasketCourtCtrl)));
}
private const string ImageContentPropertyName = "BasketCourtContent";
public static DependencyProperty CircleContentProperty = DependencyProperty.Register(ImageContentPropertyName, typeof(FrameworkElement), typeof(BasketCourtCtrl));
public FrameworkElement BasketCourtContent
{
get { return (FrameworkElement)GetValue(CircleContentProperty); }
set { SetValue(CircleContentProperty, value); }
}
#region BACKGROUND COLOR
public static DependencyProperty BasketFieldBackgroundColorProperty = DependencyProperty.Register(nameof(BasketFieldBackgroundColor), typeof(Color?), typeof(BasketCourtCtrl), new PropertyMetadata(null, OnBasketFieldBackgroundColorChanged));
public Color? BasketFieldBackgroundColor
{
get { return (Color?)GetValue(BasketFieldBackgroundColorProperty); }
set { SetValue(BasketFieldBackgroundColorProperty, value); }
}
private static void OnBasketFieldBackgroundColorChanged(DependencyObject d, DependencyPropertyChangedEventArgs e)
{
BasketCourtCtrl c = d as BasketCourtCtrl;
if (c != null)
c.OnBasketFieldBackgroundColorChanged((Color?)e.OldValue, (Color?)e.NewValue);
}
private void OnBasketFieldBackgroundColorChanged(Color? oldValue, Color? newValue)
{
this.BasketFieldBackgroundBrush = this.BasketFieldBackgroundColor.HasValue ? new SolidColorBrush(this.BasketFieldBackgroundColor.Value) : null;
}
public static DependencyProperty BasketFieldBackgroundBrushProperty = DependencyProperty.Register(nameof(BasketFieldBackgroundBrush), typeof(SolidColorBrush), typeof(BasketCourtCtrl));
public SolidColorBrush BasketFieldBackgroundBrush
{
get { return (SolidColorBrush)GetValue(BasketFieldBackgroundBrushProperty); }
private set { SetValue(BasketFieldBackgroundBrushProperty, value); }
}
#endregion BACKGROUND COLOR
#region LINE COLOR
public static DependencyProperty BasketFieldLineColorProperty = DependencyProperty.Register(nameof(BasketFieldLineColor), typeof(Color?), typeof(BasketCourtCtrl), new PropertyMetadata(null, OnBasketFieldLineColorChanged));
public Color? BasketFieldLineColor
{
get { return (Color?)GetValue(BasketFieldLineColorProperty); }
set { SetValue(BasketFieldLineColorProperty, value); }
}
private static void OnBasketFieldLineColorChanged(DependencyObject d, DependencyPropertyChangedEventArgs e)
{
BasketCourtCtrl c = d as BasketCourtCtrl;
if (c != null)
c.OnBasketFieldLineColorChanged((Color?)e.OldValue, (Color?)e.NewValue);
}
private void OnBasketFieldLineColorChanged(Color? oldValue, Color? newValue)
{
this.BasketFieldLineBrush = this.BasketFieldLineColor.HasValue ? new SolidColorBrush(this.BasketFieldLineColor.Value) : null;
}
public static DependencyProperty BasketFieldLineBrushProperty = DependencyProperty.Register(nameof(BasketFieldLineBrush), typeof(SolidColorBrush), typeof(BasketCourtCtrl));
public SolidColorBrush BasketFieldLineBrush
{
get { return (SolidColorBrush)GetValue(BasketFieldLineBrushProperty); }
private set { SetValue(BasketFieldLineBrushProperty, value); }
}
#endregion LINE COLOR
}
- Adorniamo la classe con l'attributo ContentProperty ed assegnamogli il nome della proprietà costante ImageContentPropertyName
- Deriviamo la classe dalla classe Control
- Creiamo un costruttore static ed implementiamo il metodo DefaultStyleKeyProperty.OverrideMetadata(typeof(tipo della nostra classe), new FrameworkPropertyMetadata(typeof(tipo della nostra classe)))
- Creiamo una DependencyProperty di tipo FrameworkElement con lo stesso nome dato a ImageContentPropertyName
- ... a questo punto potrebbe già essere applicabile l'immagine così come verrebbe importata
- ... se si vogliono definire delle proprietà da configurare in sviluppo, proseguiamo
- Creiamo una DependencyProperty public di tipo SolidColorBrush
- Creiamo una DependencyProperty public di tipo Color? che gestisca il colore di Background (qui chiamata BasketFieldBackgroundColor) e che richiama un PropertyMetadata(null, metodo statico per il cambio di colore)
- Creiamo il metodo statico che deve essere sollevato quando si assegna il colore
- Creiamo un metodo private non statico che determina il colore del SolidColorBrush (nel nostro esempio BasketFieldLineBrush)
|
Ora importiamo l'immagine nel nostro codice.
- Se non presente, in Esplora Soluzioni, creare la cartella Themes
- All'interno deve essere presente il file Generic.xaml. Se non presente aggiungerlo di tipo Dizionario risorse (ResourceDictionary)
- Creare un nuovo nodo Style
- Assegnare un TarteType, richiamando il nome del control appena creato (nel nostro esempio NbaLogoCtrl)
- Definire un Setter della Property Template
- Definire un Setter.Value
- Definire un ControlTemplate assegnando come TargetType sempre il nome del control
- Definire il Border
- Definire la Griglia
- Definire un Viewbox
- Creare il nodo Canvas. Assegnare un Name, Width, Height
- Inserire all'interno del Canvas la struttura XAML creata della nostra immagine
- Trovare i nodi PATH di cui si vuole gestire i colori via codice ed assegnare all'attributo Fill il Binding al Brush desiderato tra quelli creati.
<Style TargetType="{x:Type ctrls:NbaLogoCtrl}">
<Setter Property="Template">
<Setter.Value>
<ControlTemplate TargetType="{x:Type ctrls:NbaLogoCtrl}">
<Border BorderThickness="2">
<Grid Margin="3">
<Viewbox Stretch="Uniform" >
<Canvas x:Name="NbaCanvas" Width="254" Height="545" Canvas.Left="0" Canvas.Top="0" >
<Canvas.RenderTransform>
<TranslateTransform X="-11.6" Y="-11.9"/>
</Canvas.RenderTransform>
<Canvas.Resources/>
<Rectangle xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Width="276.991" Height="569.026" Name="rect32" Fill="{TemplateBinding NbaBackgroundBrush}"/>
<Path xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Name="path34" Fill="{TemplateBinding NbaLeftColorBrush}" Data="M197.43 503.7c0.497-8.029 0.331-10.678-0.754-11.985c-0.825-0.994-1.408-4.093-1.432-7.61 c-0.036-5.326-0.751-7.315-7.005-19.461c-6.674-12.964-6.914-13.672-5.728-16.952c1.106-3.059 0.949-4.088-1.469-9.664 c-2.412-5.562-3.497-6.774-9.957-11.134c-12.532-8.457-22.605-17.079-39.377-33.705c-18.725-18.567-34.272-38.982-48.689-63.936 c-5.006-8.664-5.398-9.025-9.82-9.047c-3.32-0.016-7.307-2.913-7.292-5.301c0.004-0.692-4.059-9.641-9.029-19.885 c-12.898-26.587-14.001-32.248-10.057-51.607c2.341-11.478 2.781-12.51 7.663-17.915c5.183-5.735 4.37-7.35-4.119-8.189 c-11.341-1.123-11.225-1.052-13.576-8.329c-1.156-3.579-2.102-7.635-2.102-9.013c0-4.567 5.186-19.02 12.316-34.325 c3.868-8.306 8.828-19.633 11.021-25.171c7.803-19.708 18.568-35.466 27.26-39.901c3.475-1.773 10.229-3.106 30.328-5.989 c11.188-1.605 11.976-2.418 13.583-14.014c0.935-6.744 0.842-7.801-0.924-10.661c-1.274-2.06-1.962-4.976-1.962-8.314 c0-4.694 0.263-5.277 3.021-6.703c2.666-1.378 3.021-2.085 3.021-6.017c0-9.406 1.898-14.643 7.177-19.777 c1.463-1.426 2.767-2.773 3.669-3.77H74.065c-23.482 0-42.52 19.037-42.52 42.52v433.587c0 23.482 19.037 42.52 42.52 42.52h126.485 c-0.427-0.941-1.319-2.465-2.354-3.973C195.318 535.789 195.349 537.26 197.43 503.7z"/>
<Path xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Name="path36" Fill="{TemplateBinding NbaRightColorBrush}" Data="M203.236 25.325h-36.615c0.359 0.427 0.877 0.886 1.507 1.299c4.528 2.966 11.396 10.111 12.492 12.994 c1.65 4.341 1.279 10.107-1.05 16.333c-1.338 3.574-1.901 6.772-1.523 8.656c0.712 3.561-2.786 11.11-6.926 14.95 c-1.493 1.384-3.609 4.26-4.702 6.392c-1.093 2.131-3.805 5.848-6.027 8.258c-2.22 2.41-3.888 5.152-3.703 6.093 c0.184 0.942 2.826 3.053 5.872 4.692c3.047 1.639 7.606 4.855 10.134 7.147s7.63 6.312 11.339 8.933 c13.327 9.421 19.722 25.53 19.788 49.848c0.03 10.776 5.285 36.033 9.05 43.492c5.157 10.216 7.024 15.848 8.638 26.043 c2.072 13.101 2.134 13.249 6.3 14.989c3.982 1.664 14.293 10.035 14.293 11.604c0 0.54 1.02 2.41 2.265 4.157l1.39 1.948V67.844 C245.756 44.361 226.719 25.325 203.236 25.325z M245.122 310.041c0 0.51-2.242 3.379-4.981 6.377 c-19.104 20.901-51.799 16.244-63.801-9.09c-2.904-6.132-3.207-7.672-3.207-16.335c0-8.271 0.366-10.345 2.698-15.323 c3.729-7.956 10.847-15.244 18.628-19.076c3.54-1.742 6.646-3.504 6.898-3.916c0.254-0.411-2.941-7.623-7.101-16.029 c-9.583-19.365-10.718-22.828-15.067-45.959c-1.966-10.455-3.778-19.574-4.029-20.267c-0.805-2.229-3.618-1.36-10.575 3.264 l-6.805 4.524l-6.168 15.623c-3.392 8.591-6.168 16.898-6.168 18.457c0 1.755 0.997 3.767 2.613 5.277 c4.031 3.767 5.119 6.533 11.951 30.398c5.034 17.58 6.156 22.716 5.353 24.481c-0.771 1.688-0.27 5.393 2.047 15.171 c3.408 14.388 4.679 22.013 6.268 37.606c1.611 15.83 3.315 20.844 12.486 36.75c6.097 10.572 7.969 16.944 12.25 41.694 c1.964 11.357 3.948 21.354 4.409 22.214s2.456 3.971 4.434 6.91c5.439 8.083 6.258 11.802 4.303 19.58 c-0.893 3.553-1.903 8.906-2.245 11.896c-0.687 5.984 1.479 20.477 3.938 26.365c0.831 1.987 1.282 4.482 1.005 5.546 c-0.279 1.064 0.884 6.382 2.583 11.817c5.313 16.998 5.078 24.208-1.041 31.935c-1.096 1.384-1.684 2.171-2.037 2.702 c18.392-4.684 31.995-21.354 31.995-41.204V309.186C245.386 309.323 245.122 309.653 245.122 310.041z"/>
<Path xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Name="path38" Fill="{TemplateBinding NbaBackgroundBrush}" Data="M46.676 448.242h17.189l9.916 43.835h0.166v-43.835h14.379v71.132H71.468l-10.247-43.935h-0.166v43.935 H46.676V448.242z"/>
<Path xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Name="path40" Fill="{TemplateBinding NbaBackgroundBrush}" Data="M95.768 448.242h23.223c10.991 0 16.115 7.073 16.115 17.733c0 7.571-2.728 14.346-9.256 15.939v0.199 c7.768 0.896 10.909 8.867 10.909 16.239c0 12.354-4.958 21.021-17.768 21.021H95.768V448.242z M111.139 476.137h3.72 c3.14 0 4.876-3.387 4.876-7.173c0-3.985-1.736-7.372-4.876-7.372h-3.72V476.137z M111.139 506.024h4.298 c2.81 0 5.454-2.989 5.454-8.767c0-5.479-2.645-8.568-5.454-8.568h-4.298V506.024z"/>
<Path xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Name="path42" Fill="{TemplateBinding NbaBackgroundBrush}" Data="M152.056 448.242h19.338l14.38 71.132H169.41l-1.57-12.453h-12.231l-1.569 12.453h-16.366L152.056 448.242z M161.807 462.787h-0.165l-4.215 30.187h8.595L161.807 462.787z"/>
</Canvas>
</Viewbox>
</Grid>
</Border>
</ControlTemplate>
</Setter.Value>
</Setter>
</Style>
|
Ora inseriamo l'immagine nelle nostre pagine/controlli XAML.
- Aprire lo XAML desiderato
- Nella header creare il namespace alla cartella Controls (dove sono presenti il nostri oggetti controls)
- Aggiungere (nella posizione desiderata) l'immagine creata, assegnandole le proprietà definire nell'oggetto
<Window x:Class="MatchManager.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:MatchManager"
xmlns:ctrls="clr-namespace:MatchManager.Controls"
....
WindowStartupLocation="CenterScreen">
....
....
<StackPanel>
<Canvas>
<ctrls:NbaLogoCtrl Width="180"
HorizontalAlignment="Left"
VerticalAlignment="Center"
Padding="4,0,0,0"
Canvas.ZIndex="-50"
x:Name="NbaLogo"
NbaBackgroundColor="White"
NbaLeftColor="Red"
NbaRightColor="Blue"/>
</Canvas>
</StackPanel>
|
|
|
|
|
|
|
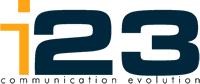 |
|
I23 di Boccaletti Emanuele 41121 Modena (MO) Tel. +39 347 1302420 emanuele@i23.eu Skype: emanuele.boccaletti |
|
|
|
|